Git - Source Code Management
Git for Developers
Configure git
git config
helps you set your email and name for your commit, we can't change the committer names after the commit. Every contributor will have their identification on every commit.
We can configure git at two levels, at the repository level, and global
# Global Config
git config --global user.name "Your Name"
git config --global user.email yourmail@domain.tld
# Repository Config - useful when you work with multiple business emails
git config --global user.name "Your Name"
git config --global user.email yourmail@domain.com
Initialize
Initialize new local repository
git init
Clone the repository
git clone [URL_FROM_GIT_SERVER]
# Examples:
git clone git@github.com:JinnaBalu/jinnabalu.github.io.git
git clone https://github.com/JinnaBalu/jinnabalu.github.io.git
Get Latest
git pull
# OR
git pull origin [BRANCH_NAME]
git status
Check the status of the files added, modified or deleted
git status
git diff
Check the lines of code added, modified ot deleted
git diff
Commit
# Commit local changes
git add --all
git commit -am "message"
# Show commits history
git log
# Show history of the file
git log -p [file]
# Who changed what and when
git blame [file]
git remote
Check the origin repository url. Update or set remote url of the local repository
# Listing remote
git remove -v
# Switch remote URL between https - ssh
git remote set-url origin [https://[url]]
git remote set-url origin [git@git://[url]]
Branch Management
# List All branches
git branch -av
# Switch between Branches
git checkout [BRANCH_NAME]
# Create a new branch
git checkout -b [BRANCH_NAME]
# Delete Branch
git branch -d [BRANCH_NAME]
# Forceful Delete
git branch -D [BRANCH_NAME]
# Delete remote branch
git branch -dr [BRANCH_NAME]
Merge changes between branches
# 1. Merging changes from feature_branch to develop
# 1.1 Get latest from develop and merge to feature_branch
git checkout develop
git pull
git checkout feature_branch
git merge --no-ff origin develop
# Note : To be safe from conflict with develop(GOOD PRACTICE), we will resolve in feature_branch
# Merge feature_branch to develop
git checkout develop
git merge --no-ff origin feature_branch
git reset | undo
Discard changes or reset back, or rebase changes
# Discard all local changes
git reset --hard HEAD
# Discard all local changes of file
git checkout HEAD [FILE_PATH]
# Soft Reset
git reset [commit_hash] --soft
# Mixed Reset
git reset [commit_hash] --mixed
git reset [commit_hash]
# Hard Reset
git reset [commit_hash] --hard
Posts on Git
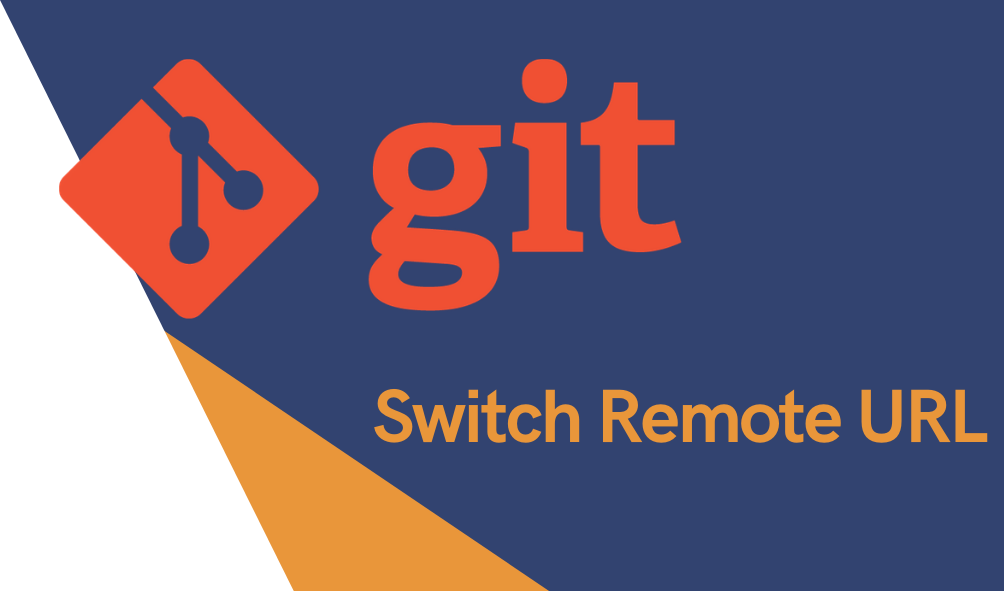
Git - Switch Remote URL
Switching remote URLs from HTTPS to SSH
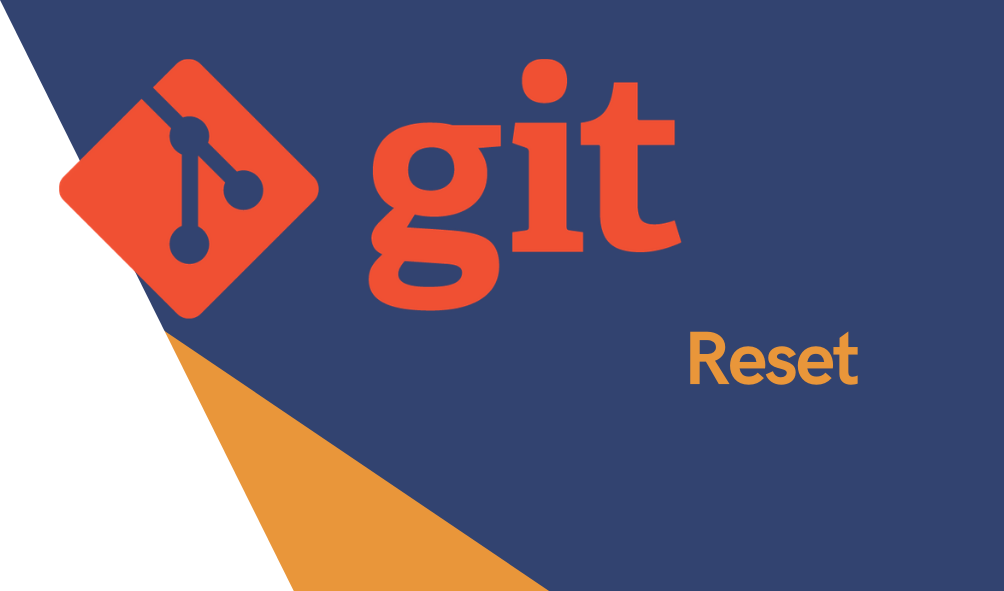
Git - Reset Commits
Remove the last commit/commits using Reset
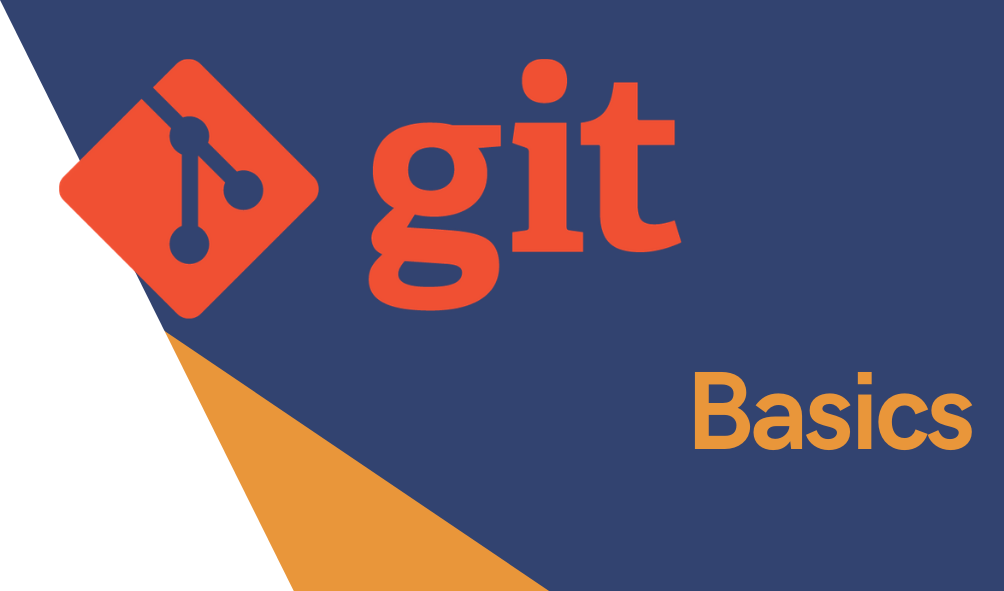
Git - Cheat Sheet
Git Cheat Sheet
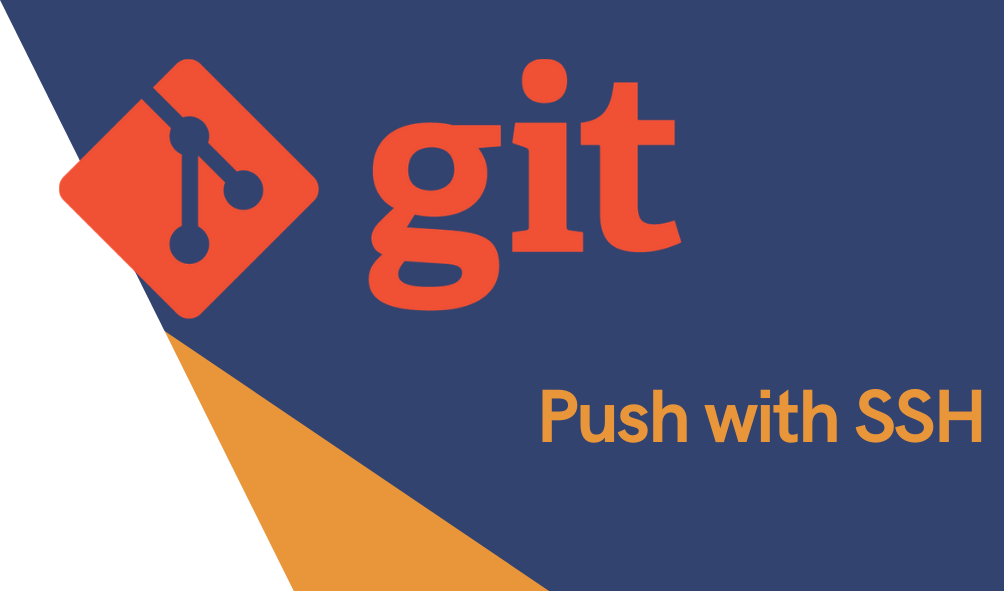
Git - Push with SSH
Setup git push with SSH
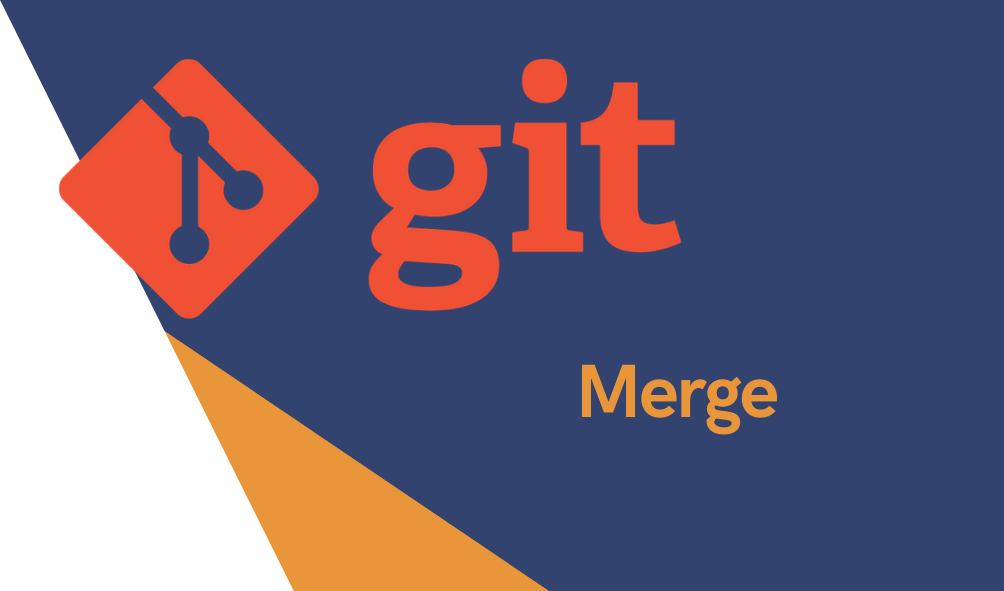
Git - Merge
GIT MERGE
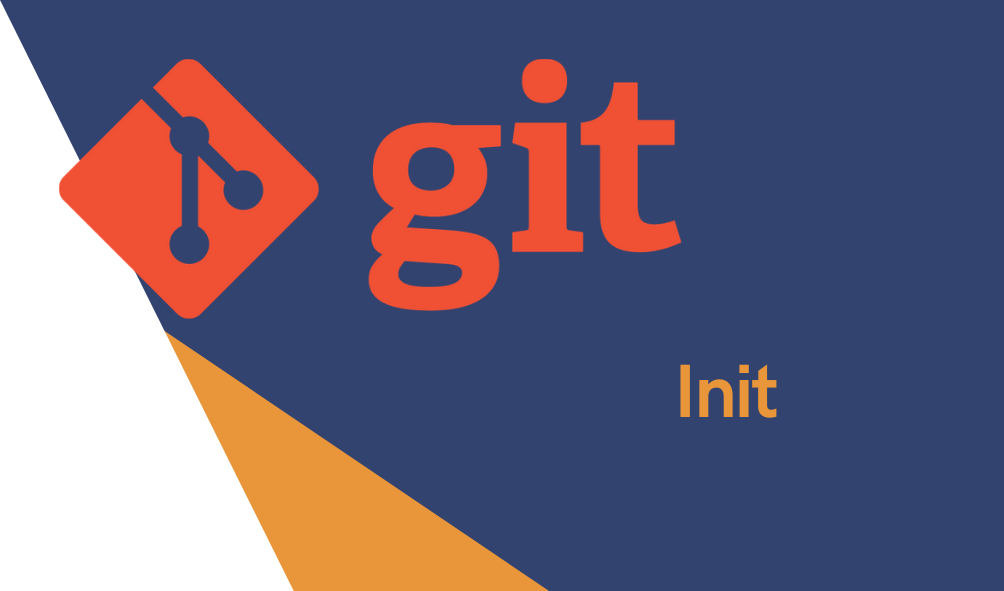
Git - Init
Git Init
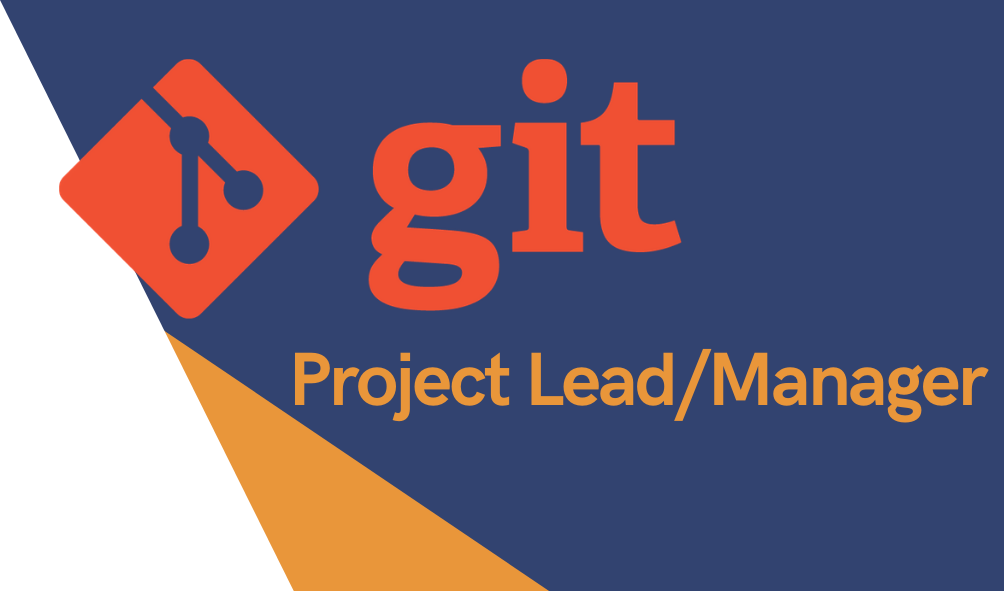
Git - Project Lead/Manager
Get count of branches
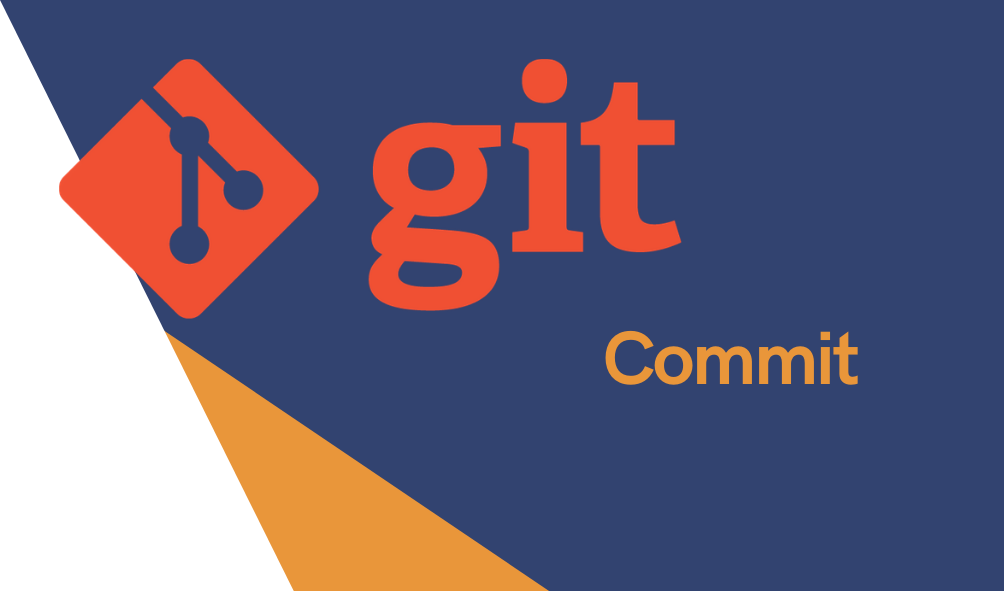
Git - Commit Files
Status
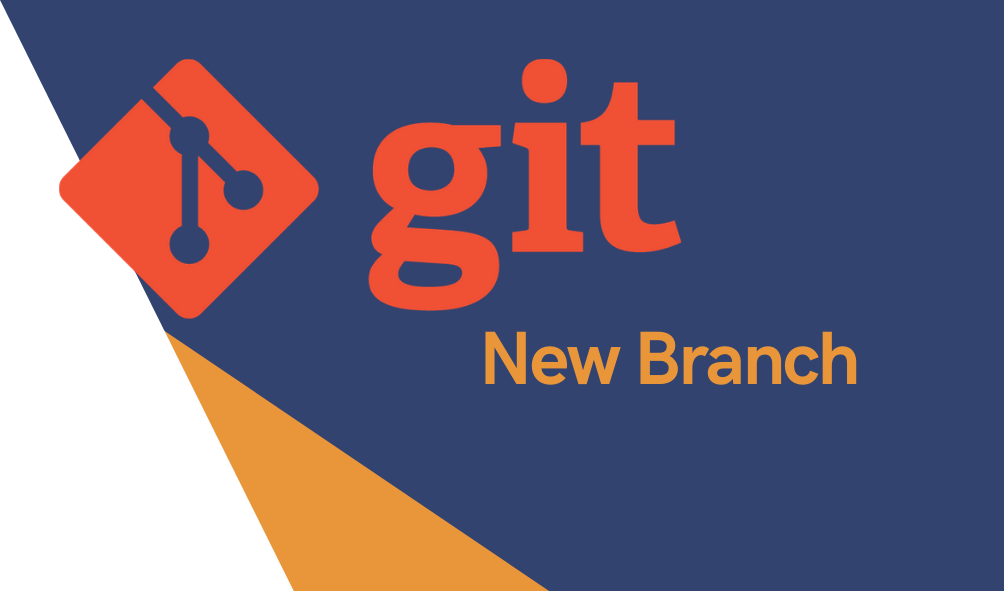
Git - Create Branch
Checkout to main branch
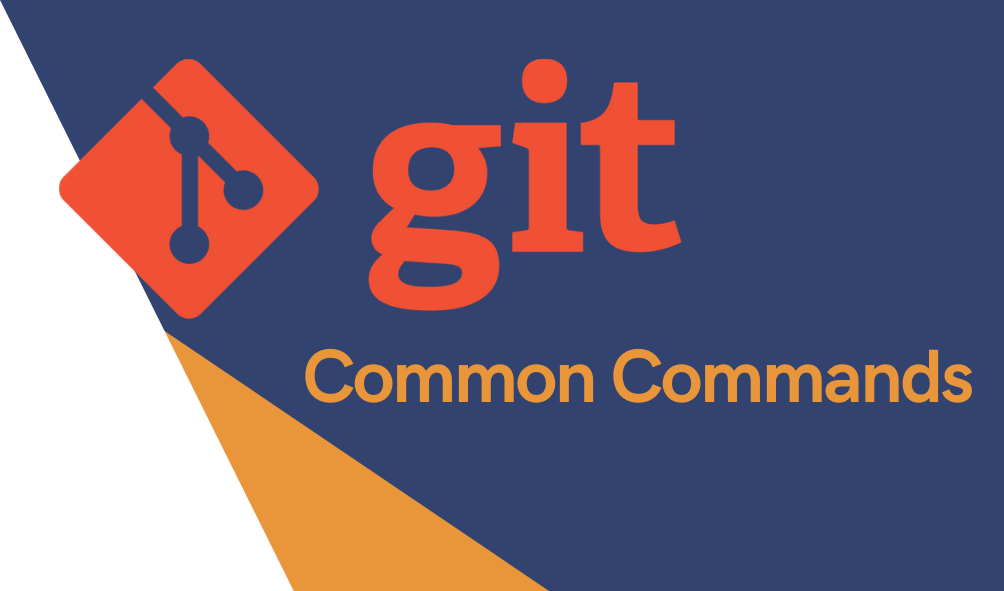
Git - Common Commands
Commit History
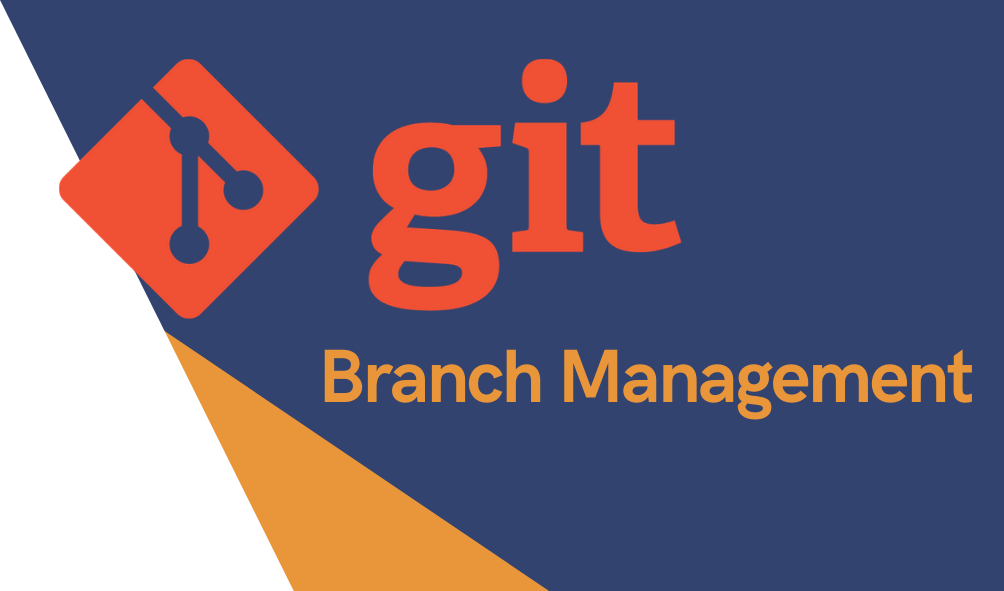
Git - Branch Management
Single branch for production and development
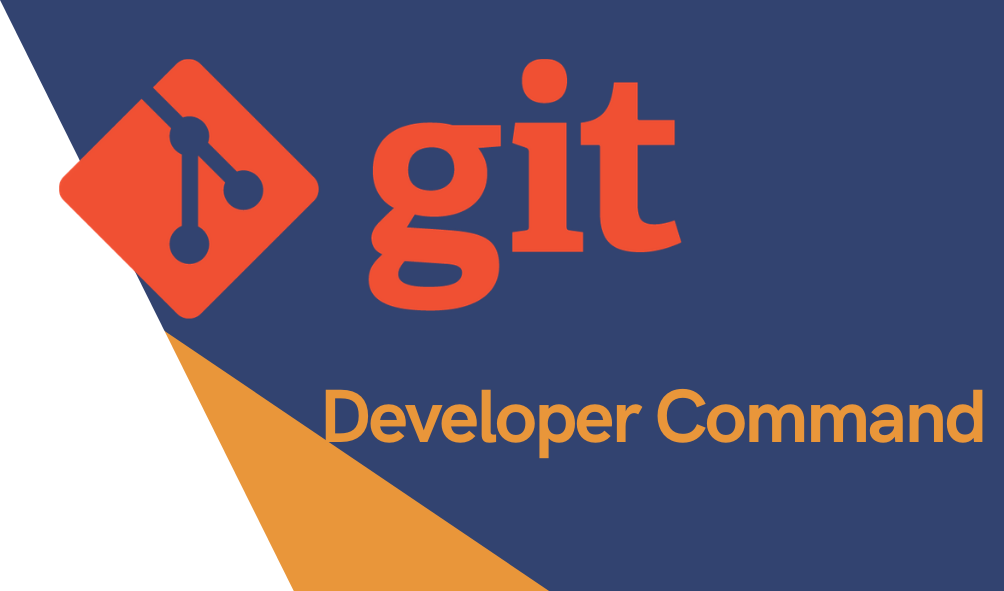
Git - Basics for a Developer
Git basics is a good idea before you start using it, as a programer upto what extent you need is the question? Let's get into it
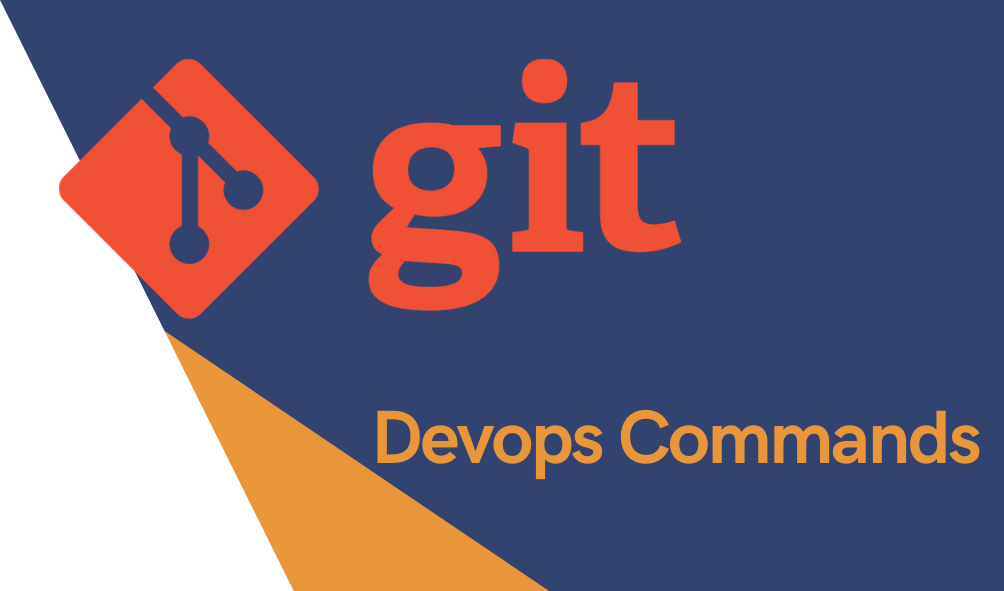
Git - Git cheat sheet | Devops Commands
Recursively remove all untracked files in the tree. git clean -f throw out all of your changes to existing files, but not new ones git reset --hard remove file from...
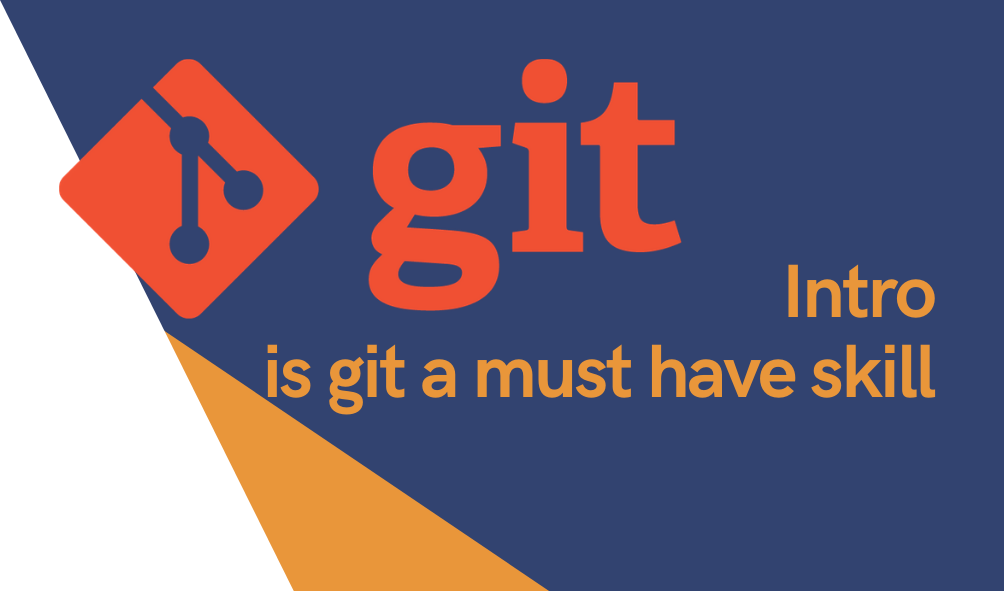
Git - Intro
There are many questions or use cases we come across in our day to day activity, like
Git for DevOps
Recursively remove all untracked files in the tree.
git clean -f
throw out all of your changes to existing files, but not new ones
git reset --hard
remove file from staging area
git rm --cached [file]
see diff of files in staging area
git diff --staged
see tracked files
git ls-files
see a branch graph
git log --graph
see all tags
git tag
see list of deleted files
git ls-files -d
restore all deleted files
git ls-files -d | xargs git checkout --
view commits not yet pushed to remote
git log --branches --not --remotes
difference between two branches
git diff --stat --color master..branch
see a list of all objects
git rev-list --objects --all
remove file from index
git rm --cached filename.txt
get a list of all commit messages for a repo
git log --pretty=format:'%s'